Published on 3/14/2025
TypeScript Hacks You Should Know As Developer
TypeScript hacks to improve code quality and productivity. Modern techniques and best practices every developer should be familiar with.
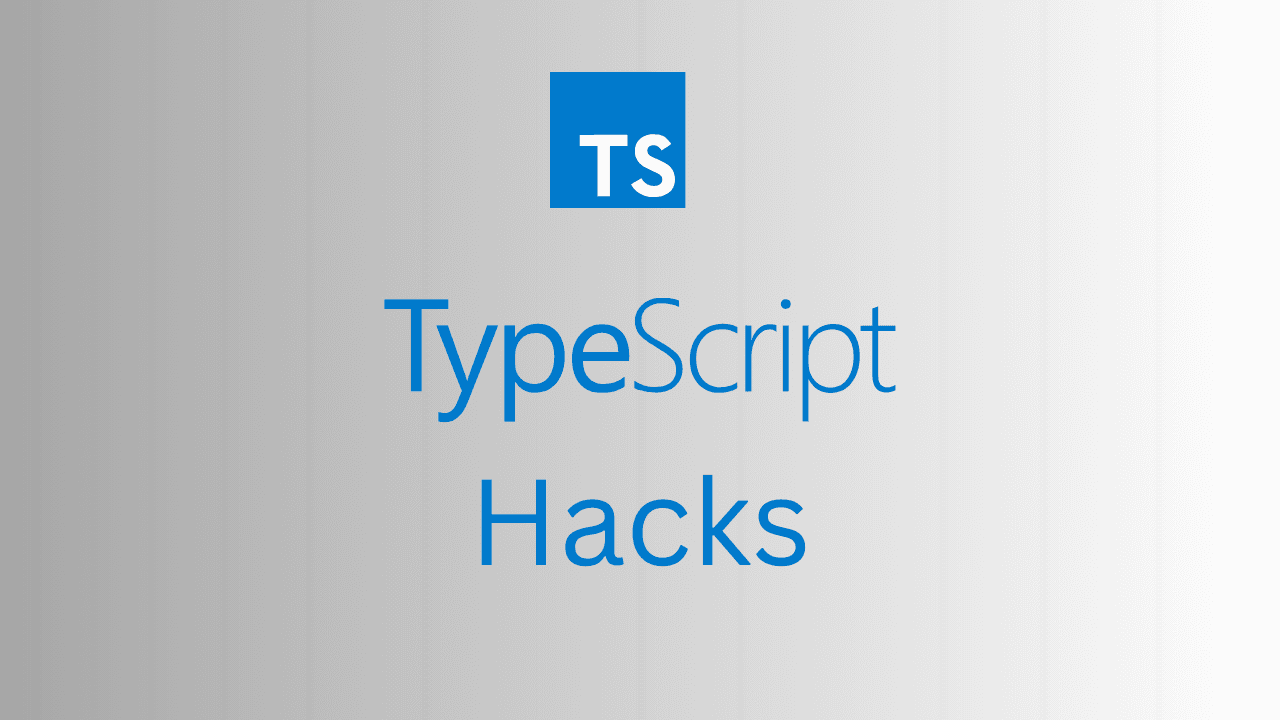
In this article I'll explain some typescript hacks. These tips will let developers produce safer, cleaner, and more effective code.
Today, TypeScript is the most common language for building websites and REST APIs. It builds on top of JavaScript by adding strong type casting and other features that make it easier to maintain and boost developer productivity.
Beyond the fundamentals, though, there are some quite effective TypeScript tricks that will advance your coding abilities. In this article, we'll look for the most useful TypeScript tricks that every developer should know.
1. Using Type Assertions for Flexible Typing
Type assertions help you tell TypeScript how to interpret a variable when you're confident about its type.
let someValue: any = "Hello, TypeScript";
let strLength: number = (someValue as string).length;
console.log(strLength); // Output: 16
This is very helpful when working with data from outside sources where TypeScript is unable to figure out the type.
2. Using the readonly modifier on immutable object (that can't be changed)
If you want to ensure that an object's properties cannot be changed after initialization, use readonly.
type User = { readonly id: number; name: string;};
const user: User = { id: 1, name: "Kaushal" };
user.name = "Doe"; // Allowed
user.id = 2; // Error: Cannot assign to 'id' because it is a read-only
3. Strict Object Keys with Record
The Record utility type can be used to define an object with specific keys and types on the fly.
type UserRole = "admin" | "editor" | "viewer";
type Permissions = Record;
const userPermissions: Permissions = {
admin: ["create", "delete", "update"],
editor: ["update"],
viewer: ["read"]
};
This guarantees that userPermissions only allow valid keys (admin, editor, viewer) to be utilized.
4. Utility Types for Reusing Code
TypeScript provides built-in utility types that make working with types easier. Some useful ones include:
- Partial<T> - Makes all properties optional.
- Required<T> - Makes all properties required.
- Pick<T, K> - Picks specific properties from a type.
- Omit<T, K>- Omits specific properties from a type.
type User = {
id: number;
name: string;
};
type PartialUser = Partial<User>; // All properties optional
const user: PartialUser = { name: "Kaushal" };
5. Type Guards for Better Type Safety
Type guards allow you to check types at runtime.
function isString(value: unknown): value is string {
return typeof value === "string";
}
function printLength(value: unknown) {
if (isString(value)) {
console.log(value.length); // TypeScript knows 'value' is a string
}
}
6. Exhaustive Type Checking with never
The never type is useful for ensuring exhaustive checks in switch cases.
type Color = "red" | "green" | "blue";
function getColorCode(color: Color) {
switch (color) {
case "red": return "#FF0000";
case "green": return "#00FF00";
case "blue": return "#0000FF";
default:
const _exhaustiveCheck: never = color;
throw new Error("Unexpected color: " + color);
}
}
This ensures all possible values of Color are handled.
7.Conditional Types for Advanced Type Logic
Conditional types allow you to create types that depend on other types.
type IsString<T> = T extends string ? "Yes" : "No";
type Check = IsString<number>; // Output: 'No'
let no: Check = 'No';
let yes: Check = 'Yes';
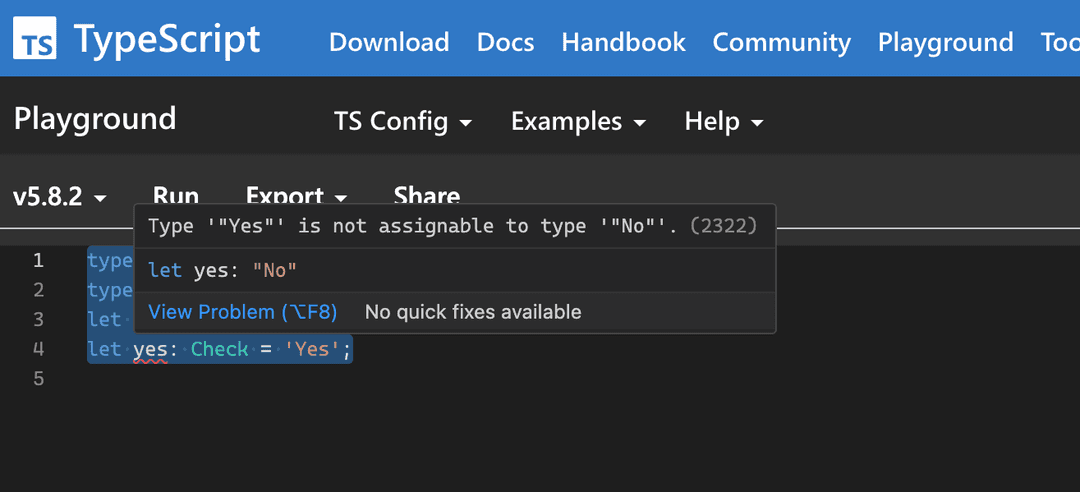
This is helpful for creating flexible and reusable types.
8. Union Types for Multiple Types
Union types allow you to define a type that can be one of several types. This is useful for functions that can accept multiple types of arguments.
function printId(id: number | string) {
console.log(`ID: ${id}`);
}
printId(101); // OK
printId("202"); // OK
9.Use Non-null Assertion Operator (!)
When you're sure that a value is not null or undefined, you can use the non-null assertion operator (!) to tell TypeScript to ignore those types.
let someValue: any = "Hello, TypeScript!";
let strLength: number = someValue!.length;
console.log(strLength); // Output: 16
function getValue(): string | null {
return 'Hello';
}
const value = getValue()!; // Asserts that value is not null
console.log(value.toUpperCase()); // No error
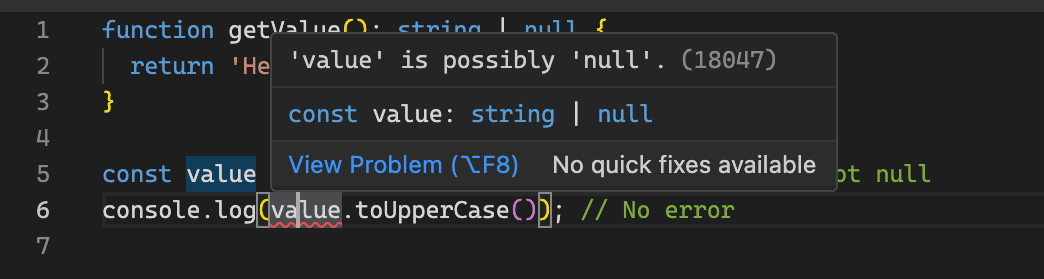
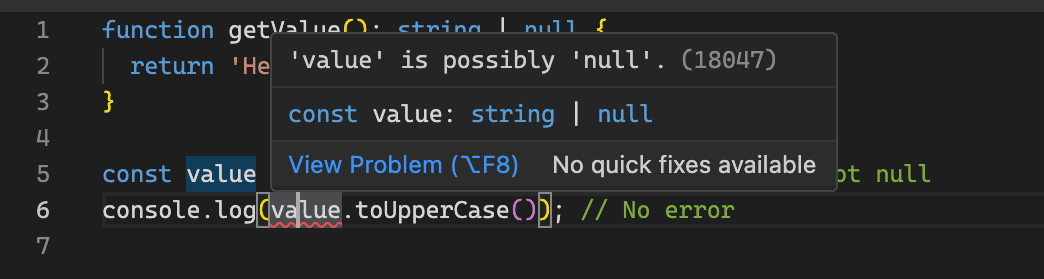
Conclusion
They will help you write code that is cleaner, safer, and easier to maintain. These tips will help you get the most out of TypeScript and make your work flow better, no matter how much experience you have as a developer.